For you and the copiers of your Trades, for free
API Trading allows you to create a Trade from your own computer program. We will see in this tutorial how to create a trade on major cryptocurrency exchanges like Binance Futures, Binance Spot, Huobi, Crypto.com, Kraken, Kucoin, Bittrex, Bitfinex, OKX or Ascendex and Trading Bot.
Thanks to our partnership with the main exchange platforms, like Huobi, it’s totally free. We are indeed a licensed broker/broker. Thus, they automatically pay us part of the trading fees. It does not change anything for you! On Binance Spot and Binance Futures, there is one condition: you must use a Binance account created after March 2020 and without a referral link.
If you want replicate your trades on the accounts of your subscribers, it is quite possible even with this method of API Trading. We will see how to create your Copy Trading group. We have other methods than the API Trading to create Trades like our Smart Trading Terminal suitable for Manual Trading.
For this API Trading method, you can code in any computer language.
API Trading: setting up your account
1) Create an account on Wall of Traders.
2) Connect by clicking on “Stay connected”:
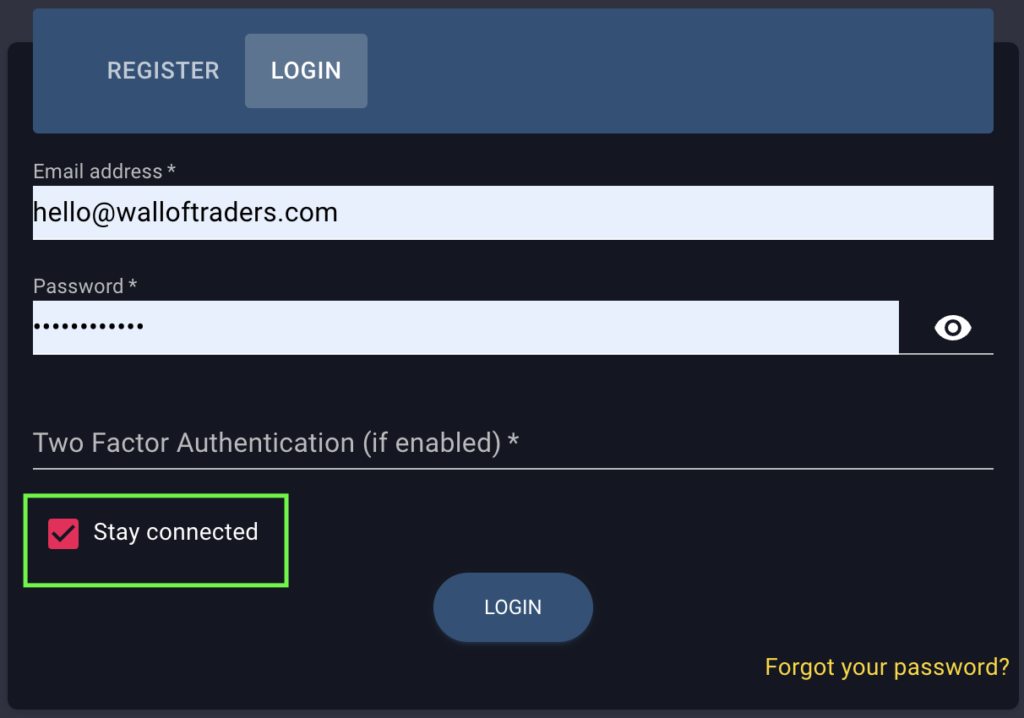
3) Switch to Developer mode : right click anywhere on the page then Inspect, or press F12 :

Then click on Traders in the Menu on the left, it refreshes the data. Then in the DevTools on the right: Click on “Network”, then on “Groups”, and scroll down to “authorization: Bearer ………” :

Copy the characters after “Bearer”; in my example from eyJhbG….. to ….. 66qAkz4. Save this value somewhere. We will use it in {Token} in the code.
4) Create an API on the exchange of your choice, for example on Binance. Save the API Key and API Secret.
5) Then on your Wall Of Traders account, add the API Key and API Secret of this exchange.
6) Then modify this exchange by clicking on the pencil:

In the URL you will see https://walloftraders.com/exchanges/12345 where 12345 is the {ExchangeId}. Copy this number and save it. It will be used in {ExchangeId} in the code.
7) On the Traders page, click at the top right on “CREATE A TRADER“. When you will validate the form, you will be redirected to a page. In the URL you will see https://walloftraders.com/manageFollowers/56789 where 56789 is the {GroupId}. Copy this number and save it. It will be used in {GroupId} in the code.
Preparation is complete! Now all you have to do is Coding using the code below.
API Trading: how to create a Trade
It’s your turn by copying and pasting the code below and adapting it to your programming language:
POST https://api2.walloftraders.com/api/v1/Order
Header:
Authorization: 'Bearer {Token}',
Content-Type: 'application/json',
Body:
{
"isBuy": true, //true: Buy/Long mode - false: Sell/Short mode
"accountId": {ExchangeId},
"pair": "BTC-USDT",
"groupId": {GroupId},
"leverage": 1, //Add this line only if the exchange you are trading on uses leverage. For example add it for Binance Futures but remove it for Binance Spot.
"pourcentage": 5, //Percentage of capital for the Trade. Between 0.01 and 100.
"startPrice": 19000, //Limit entry price. Put 0 to enter the market.
"targets": [ //The sum of the "percentages" must not be greater than 100.
{
"pourcentage": 50, //Share in % to close for this Take Profit. For example, put 100 for a single Take Profit, or 50 to close the half.
"orderType": 0, //0: limit order - 1: market order.
"price": null, //Take Profit price, if "price" != null, the next line "profit" is not read.
"profit": 2, //If "price" == null, the target price will be equal to startPrice*(1+-profit/100).
"trailing": null, //Trailing percentage (optional line).
}
],
"stopPrice": 18000, //Put null if you don't want to put Stop Loss.
"stopProfit": -2, //If stopPrice == null, then we automatically calculate the Stop price = startPrice-stopProfit/100 (optional line)
"orderId": 987654, //To update the Trade, insert the orderId here (optional line)
//Other optional features
"note": "Blabla", //Free text
"dontDoOrder": false, //Doesn't create the Trade for me, but creates it for the copiers. Only if GroupId defined.
"dontDoStart": false, //Do not create entry but create TP and SL.
"timeOutStart": null,
"timeOutStopLoss": null,
"trailingStart": null,
"startTriggerPrice": null,
"startTradingViewGuid": null,
"trailingStopLoss": null,
"orderTypeStop": 0,
"MoveSL": 0, //SL moves: if 0 to entry price when TP1 is reached, if 1 to TP1 when TP2 is reached, if 2 to all (entry price and TPn) when TPn+1 is reached.
}
To delete a Trade, i.e. cancel pending entries, and delete TP and SL, but not close the Trade:
api/v1/Order/${orderId}, {
method: 'DELETE',
}
To close a trade, i.e. remove the TP and SL and also resell the bought cryptos (or buy them back in the event of a sale/short):
api/v1/Order/PanicSell?orderId=${orderId}&pourcentage=${pourcentage},
{
method: 'POST',
},
Where ${percentage} must be equal to 100 if you want to close the entire position, but can for example be equal to 50 to close only half.
API Trading: program example in Python
import logging
import typing
logger = logging.getLogger()
import time
import hmac
from urllib.parse import urlencode
import hashlib
import requests
import json
from datetime import datetime
import time
import requests
import pandas as pd
import logging
import json
from datetime import datetime
import time
import requests
import pandas as pd
import logging
import logging
import datetime
import typing
logger = logging.getLogger()
import time
import hmac
from urllib.parse import urlencode
import hashlib
import requests
import json
import random
import json
from datetime import datetime
import time
import requests
import pandas as pd
import logging
def place_order_buy( q) :
while True:
url = 'https://api2.walloftraders.com/api/v1/Order'
payload={
"isBuy": True,
"accountId": 12345,
"pair": "BTC-USDT",
"groupId": 12345,
"leverage": 100,
"pourcentage": q,
"startPrice": 0,
"targets": [],
}
headers = {'Authorization': 'Bearer xxx',
'Content-Type': 'application/json'}
order_status = requests.post(url,json=payload,headers=headers)
if order_status is not None:
break
return order_status
def place_order_sell( q) :
while True:
url = 'https://api2.walloftraders.com/api/v1/Order'
payload={
"isBuy": False,
"accountId": 12345,
"pair": "BTC-USDT",
"groupId": 12345,
"leverage": 100,
"MoveSL": 0,
"pourcentage": q,
"startPrice": 0,
"targets": [],
}
headers = {'Authorization': 'Bearer xxx',
'Content-Type': 'application/json'}
order_status = requests.post(url,json=payload,headers=headers)
if order_status is not None:
break
return order_status
def place_order_buy2( q, tp, sl) :
while True:
url = 'https://api2.walloftraders.com/api/v1/Order'
payload={
"isBuy": True,
"accountId": 12345,
"pair": "BTC-USDT",
"groupId": 12345,
"leverage": 100,
"pourcentage": q,
"startPrice": 0,
"targets": [
{
"pourcentage":100,
"orderType":0,
"price": tp,
}
],
"stopPrice": sl,
}
headers = {'Authorization': 'Bearer xxx',
'Content-Type': 'application/json'}
order_status = requests.post(url,json=payload,headers=headers)
if order_status is not None:
break
return order_status
def place_order_sell2( q, tp, sl) :
while True:
url = 'https://api2.walloftraders.com/api/v1/Order'
payload={
"isBuy": False,
"accountId": 12345,
"pair": "BTC-USDT",
"groupId": 12345,
"leverage": 100,
"MoveSL": 0,
"pourcentage": q,
"startPrice": 0,
"targets": [
{
"pourcentage":100,
"orderType":0,
"price": tp,
}
],
"stopPrice": sl,
}
headers = {'Authorization': 'Bearer xxx',
'Content-Type': 'application/json'}
order_status = requests.post(url,json=payload,headers=headers)
if order_status is not None:
break
return order_status
def close_order_ID( ID) :
while True:
url = 'https://api2.walloftraders.com/api/v1/Order/PanicSell?orderID='+str(ID)+'&pourcentage=100'
headers = {'Authorization': 'Bearer xxx',
'Content-Type': 'application/json'}
payload={
"accountId": 12345,
"pair": "BTC-USDT",
"groupId": 12345,
"leverage": 100,
}
order_status = requests.post(url,json=payload,headers=headers)
if order_status is not None:
# print(order_status)
break
#if order_status is not None:
# print('ordin deschis')
return order_status
I hope this article has you more! If you have any questions, you can talk to us directly on Telegram.
This article is not investment advice. Do your own research before investing in the cryptocurrency market.